前回の続きです。
ファイル構成と今回のゴール
⚫️ファイル構成
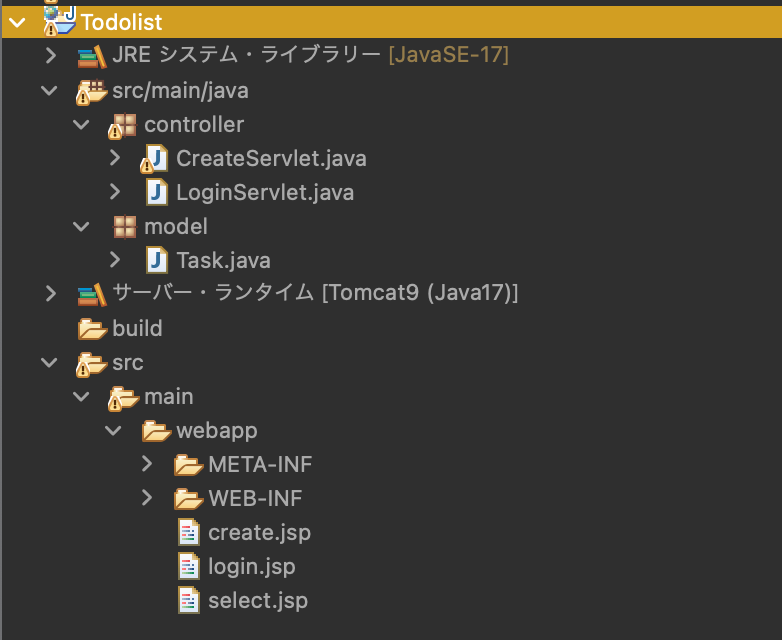
⚫️今回のゴール
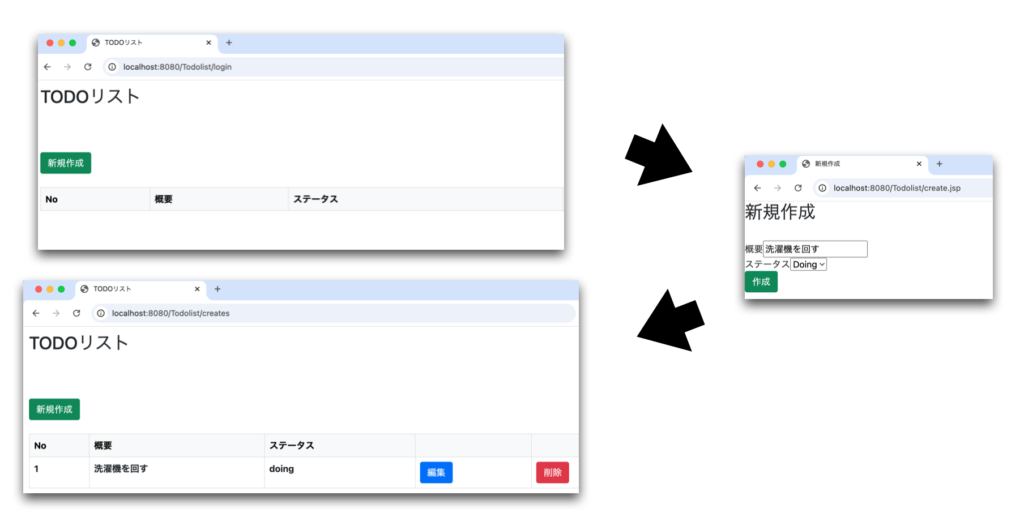
実装
package controller;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import model.Task;
@WebServlet("/creates")
public class CreateServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
String summary = request.getParameter("summary");
String status = request.getParameter("status");
Task newTask = new Task(summary, status);
HttpSession session = request.getSession();
List<Task> todoList = (List<Task>) session.getAttribute("todoList");
// もしリストがまだ作成されていなければ、新しいリストを作成
if (todoList == null) {
todoList = new ArrayList<>();
}
// 新しいタスクをリストに追加
todoList.add(newTask);
// 更新されたリストをセッションに保存
session.setAttribute("todoList", todoList);
RequestDispatcher req = request.getRequestDispatcher("select.jsp");
req.forward(request,response);
}
}
package model;
public class Task {
//概要
private String summary;
//ステータス
private String status;
public Task(String summary, String status) {
this.summary = summary;
this.status = status;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="javax.servlet.http.HttpSession" %>
<%@ page import="model.Task" %>
<%@ page import="java.util.ArrayList" %>
<%@ page import="java.util.List" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>TODOリスト</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
</head>
<body>
<div style="padding: 10px">
<h2>TODOリスト</h2>
<br>
<br>
<br>
<button type="button" class="btn btn-success" onclick="location.href='./create.jsp'">新規作成</button>
<br>
<br>
<table class="table table-bordered">
<thead class="table-light">
<tr>
<th scope="col">No</th>
<th scope="col">概要</th>
<th scope="col">ステータス</th>
<th scope="col"></th>
<th scope="col"></th>
</tr>
</thead>
<%
List<Task> todoList = (List<Task>) session.getAttribute("todoList");
if(todoList != null){
for(int i = 0;todoList.size() > i;i++){
Task task = todoList.get(i);
%>
<tbody>
<tr>
<th scope="col"><%=i + 1 %></th>
<th scope="col"><%=task.getSummary() %></th>
<th scope="col"><%=task.getStatus() %></th>
<th scope="col">
<form action="edit" method="post">
<button type="submit" class="btn btn-primary">編集</button>
</form>
</th>
<th scope="col">
<form action="delete" method="post">
<button type="submit" class="btn btn-danger">削除</button>
</form>
</th>
</tr>
</tbody>
<%
}
}
%>
</table>
</div>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>新規作成</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
</head>
<body>
<h2>新規作成</h2><br>
<form action="creates" method="post">
概要<input type="textarea" name="summary" rows="3"></textarea><br>
ステータス<select name="status" id="">
<option value="todo">Todo</option>
<option value="doing">Doing</option>
<option value="done">Done</option>
</select><br>
<input type="submit" class="btn btn-success" value="作成"></input>
</form>
</body>
</html>